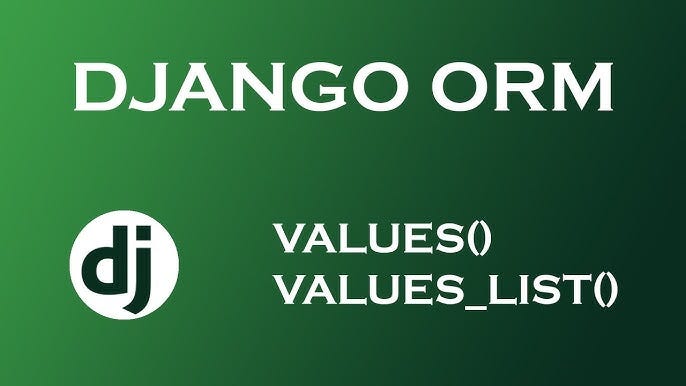
Understanding values()
vs values_list()
When working with Django ORM, you often need to extract specific fields from your database. Two popular methods for this are values()
and values_list()
. While they seem similar, they serve different purposes and return data in different formats. Let’s break down their differences to help you choose the right one for your use case.
1. values()
Method
The values()
method returns a QuerySet of dictionaries, where each dictionary contains key-value pairs representing field names and their respective values.
Use Case:
When you need key-value pairs for easier access by field names (e.g., in APIs, JSON responses).
Example:
# Fetching specific fields using values()
products = Product.objects.values('name', 'price')
print(products)
Output:
[
{"name": "T-Shirt", "price": 500},
{"name": "Jeans", "price": 1200}
]
2. values_list()
Method
The values_list()
method returns a QuerySet of tuples, with each tuple containing the field values in the order they are specified.
Use Case:
When you need simpler, lightweight data structures, such as lists or tuples, to reduce overhead.
Example:
# Fetching specific fields using values_list()
products = Product.objects.values_list('name', 'price')
print(products)
Output:
[('T-Shirt', 500), ('Jeans', 1200)]
3. flat=True
in values_list()
By using flat=True
, you can flatten the result into a single list if you're fetching only one field.
Example:
# Fetching only product names
product_names = Product.objects.values_list('name', flat=True)
print(product_names)
Output:
['T-Shirt', 'Jeans']
4. named=True
in values_list()
(Django 3.1+)
If you want more readable and self-explanatory results, use named=True
. This returns a namedtuple, allowing you to access values by field names instead of index positions.
Example:
# Using named=True for clearer access
products = Product.objects.values_list('id', 'name', 'price', named=True)
for product in products:
print(f"ID: {product.id}, Name: {product.name}, Price: {product.price}")
Output:
ID: 1, Name: T-Shirt, Price: 500
ID: 2, Name: Jeans, Price: 1200
Key Differences at a Glance
Feature values()
values_list()
Return Type QuerySet of dictionaries QuerySet of tuples or list Field Access By field names (key-value) By index or field names (named=True
) Use Case Ideal for APIs and JSON responses Suitable for lightweight data handling Customization Not customizable by structure Easily manipulated with flat
or named
Which One Should You Use?
values()
: Best for scenarios where you need to work with field names directly, like API responses or dynamic templates.values_list()
: Perfect for simpler, more efficient data handling, especially when you don’t need the overhead of dictionaries.
By understanding these differences, you can optimize your Django queries for better performance and cleaner code!